1、首页首先设置数据库:表名叫good,表的信息如下图: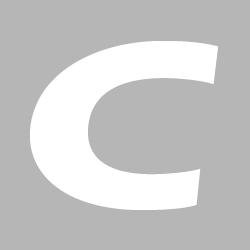
插入信息为: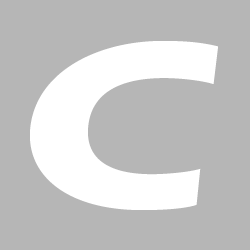
2、展示项目结构:

3、第一步写jsp页面,也就是mvc模式的view视图层:
<%@ page language="java" import="java.util.*" pageEncoding="UTF-8"%>
<%@ taglib uri="http://java.sun.com/jsp/jstl/core" prefix="c"%>
<%
String path = request.getContextPath();
String basePath = request.getScheme()+"://"+request.getServerName()+":"+request.getServerPort()+path+"/";
%>
<!DOCTYPE HTML PUBLIC "-//W3C//DTD HTML 4.01 Transitional//EN">
<html>
<head>
<base href="<%=basePath%>">
<title>My JSP 'index.jsp' starting page</title>
<meta http-equiv="pragma" content="no-cache">
<meta http-equiv="cache-control" content="no-cache">
<meta http-equiv="expires" content="0">
<meta http-equiv="keywords" content="keyword1,keyword2,keyword3">
<meta http-equiv="description" content="This is my page">
<!--
<link rel="stylesheet" type="text/css" href="styles.css">
-->
</head>
<body>
<form action="./good.do">
<table width="600" border="2" cellspacing="0" cellpadding="0"
height="10">
<tr>
<td width="120" height="10" align="center">
商品名称
</td>
<td width="120" height="30" align="center">
商品类型
</td>
<td width="120" height="30" align="center">
商品价格
</td>
<td width="120" height="30" align="center">
库存数量
</td>
<td width="120" height="30" align="center">
制造商
</td>
</tr>
<c:forEach var="good" items="${goodlist}">
<tr>
<td width="120" height="10" align="center">
${good.name}
</td>
<td width="120" height="10" align="center">
${good.model}
</td>
<td width="120" height="10" align="center">
${good.price}
</td>
<td width="120" height="10" align="center">
${good.number}
</td>
<td width="120" height="10" align="center">
${good.maker}
</td>
</tr>
</c:forEach>
</table>
<a href="./good.do?currentpage=${1}">首页</a>
<a href="./good.do?currentpage=${currentpage-1}">上一页</a>
<a href="./good.do?currentpage=${currentpage+1}">下一页</a>
<a href="./good.do?currentpage=${pagecount}">尾页</a>
<br>
共有${pagecount} 页,当前页是${currentpage},共有${total}个商品
</form>
</body>
</html>
3、接下来写model层:
建一个javabean代码如下:
package cn.csdn.web.domain;
import java.io.Serializable;
public class Good implements Serializable {
private Integer id;
private String name;
private String model;
private Integer price;
private Integer number;
private String maker;
public Good() {
super();
// TODO Auto-generated constructor stub
}
public Integer getId() {
return id;
}
public void setId(Integer id) {
this.id = id;
}
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
public String getModel() {
return model;
}
public void setModel(String model) {
this.model = model;
}
public Integer getPrice() {
return price;
}
public void setPrice(Integer price) {
this.price = price;
}
public Integer getNumber() {
return number;
}
public void setNumber(Integer number) {
this.number = number;
}
public String getMaker() {
return maker;
}
public void setMaker(String maker) {
this.maker = maker;
}
}
接下来是写一些方法在一个接口中:
package cn.csdn.web.dao;
import java.util.List;
import cn.csdn.web.domain.Good;
public interface GoodDao {
List<Good> select(Integer nowpage);
int pageCount();
}
最后实现这些方法:
package cn.csdn.web.dao;
import java.sql.Connection;
import java.sql.DriverManager;
import java.sql.PreparedStatement;
import java.sql.ResultSet;
import java.sql.SQLException;
import java.util.ArrayList;
import java.util.List;
import cn.csdn.web.domain.Good;
public class GoodDaoImpl implements GoodDao {
int pagesize = 3;
public static int total = 0;
private static Connection conn = null;
private PreparedStatement pstmt = null;
private ResultSet rs = null;
private static final String URL = "jdbc:mysql://localhost:3306/3g?user=root&password=123&useUnicode=true&characterEncoding=UTF-8";
static {
try {
Class.forName("com.mysql.jdbc.Driver");
try {
conn = DriverManager.getConnection(URL);
} catch (SQLException e) {
// TODO Auto-generated catch block
e.printStackTrace();
}
} catch (ClassNotFoundException e) {
// TODO Auto-generated catch block
e.printStackTrace();
}
}
private void release(ResultSet rs, PreparedStatement pstmt) {
if (rs != null) {
try {
rs.close();
} catch (SQLException e) {
e.printStackTrace();
}
}
if (pstmt != null) {
try {
pstmt.close();
} catch (SQLException e) {
e.printStackTrace();
}
}
}
public List<Good> select(Integer nowpage) {
List<Good> lists = new ArrayList<Good>();
String sql = "select id,name,model,price,number,maker from good limit ?,?";
try {
pstmt = conn.prepareStatement(sql);
int index = 1;
pstmt.setInt(index++, (nowpage - 1) * pagesize);
pstmt.setInt(index++, pagesize);
rs = pstmt.executeQuery();
while (rs.next()) {
Good entity = new Good();
entity.setId(rs.getInt("id"));
entity.setName(rs.getString("name"));
entity.setModel(rs.getString("model"));
entity.setPrice(rs.getInt("price"));
entity.setNumber(rs.getInt("number"));
entity.setMaker(rs.getString("maker"));
lists.add(entity);
}
} catch (SQLException e) {
e.printStackTrace();
}
release(rs, pstmt);
return lists;
}
public int pageCount() {
int pageCount = 0;
String sql = "select count(*) from good";
try {
pstmt = conn.prepareStatement(sql);
rs = pstmt.executeQuery();
if (rs.next()) {
total = rs.getInt(1);
pageCount = (total - 1) / pagesize + 1;
}
} catch (SQLException e) {
// TODO Auto-generated catch block
e.printStackTrace();
}
return pageCount;
}
}
5、最后实现mvc中的控制层control:
package cn.csdn.web.servlet;
import java.io.IOException;
import java.util.List;
import javax.servlet.ServletException;
import javax.servlet.http.HttpServlet;
import javax.servlet.http.HttpServletRequest;
import javax.servlet.http.HttpServletResponse;
import cn.csdn.web.dao.GoodDao;
import cn.csdn.web.dao.GoodDaoImpl;
import cn.csdn.web.domain.Good;
public class GoodServlet extends HttpServlet {
public void doGet(HttpServletRequest request, HttpServletResponse response)
throws ServletException, IOException {
request.setCharacterEncoding("UTF-8");
response.setCharacterEncoding("UTF-8");
int currentpage = 1;
GoodDao gDao = new GoodDaoImpl();
int pagecount = gDao.pageCount();
String page = request.getParameter("currentpage");
if (page != null) {
currentpage = Integer.parseInt(page);
if (currentpage <= 1) {
currentpage = 1;
}
if (currentpage >= pagecount) {
currentpage = pagecount;
}
}
List<Good> GoodList = gDao.select(currentpage);
request.setAttribute("goodlist", GoodList);
request.setAttribute("currentpage", currentpage);
request.setAttribute("pagecount", pagecount);
// 总条数
int total = GoodDaoImpl.total;
request.setAttribute("total", total);
request.getRequestDispatcher("./index.jsp").forward(request, response);
}
public void doPost(HttpServletRequest request, HttpServletResponse response)
throws ServletException, IOException {
this.doGet(request, response);
}
}
6、最后是实现的效果图:

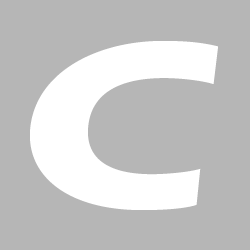
分享到:
相关推荐
JSP+JavaBean+servlet实现的分页,在网上找到的一起分享吧 已经降低积分了
使用JSP+JavaBean+Servlet实现数据分页 附带有数据库建表脚本 下载后可以直接运行、运行login.jsp 无需用户名和密码 直接登陆即可!
用jsp+javabean+servlet实现的分页 数据库用的mysql
文章整理: www.diybl.com 文章来源: 网络 去论坛 建我的blog 今天给同学用JSP+JavaBean+Servlet做了个分页。用的是MySQL+Tomcat+MyEclispe环境。
本分页系统采用JSP+JavaBean+Servlet实现。环境:Mysql+Tomcat+MyEclipse
jsp+javabean+servlet使用简单的MVC模式实现登录注册、留言板、分页的功能。model(javabean)层实现读写数据库,数据库名为webapp,没有密码;view(JSP)实现展示信息;controller(servlet)层联系model和view,...
jsp+javabean实现的分页web程序,另外附上我对这个程序做了个总结 和程序所需要用到的数据库,希望对大家有帮助。
jsp+javabean+servlet相结合的分页模式,是个很好的分页典型例子!
jsp+servlet+javabean实现登陆、分页功能
这是我前段时间写的一个分页,是用Jsp+Javabean+Servlet对Oracle 和Mysql分页
自己根据所作的一个项目对mysql分页的总结,菜鸟还有理解不到位的地方还请老鸟指教,因为自己纯手工制作所以分值高点犒劳一下自己。网上也有很多类似的东西,我想应该和我的不同
JavaBean+Servlet+jsp实现分页显示(原创)
基于Jsp+JavaBean+Servlet实现MVC模式的分页模板 分页没有css,但分页功能齐全 对初学者应该有帮助 分三层编写dao层 service层 web层 数据库可以用ms server2005 或者 mysql5.0 脚本为ms2005.sql 、mysql.sql 切换...
用jsp+JavaBean+Servlet实现的分页,有不足之处请指教
利用servlet+jsp+javabean进行分页查询,用到的数据库为mysql
5.Servlet + JSP +JavaBean + JDBC(DBUtils)+ mysql 6.数据库 create database day19; use day19; create table t_customer( id varchar(40) primary key, username varchar(20), gender varchar(10), ...
这是一个jsp+javaBean+servlet分页 主要功能 是通过 jstl标签中的 中的开始 begin和末尾end、list结果集的items属性 操作整个分页。大家有兴趣看看
jsp+Servlet+JavaBean分页的两种写法
jsp+javabean+servlet+ajax,数据分页显示,使用SERVLET完成增删改查操作, 文件、图片上传,使用smartUpload组件完成上传操作。 MVC模式 非常适合初学者;
一个简单实用的jsp+servlet+javabean 分页