在Android的触摸消息中,已经实现了三种监测,它们分别是
1)pre-pressed:对应的语义是用户轻触(tap)了屏幕
2)pressed:对应的语义是用户点击(press)了屏幕
3)longpressed:对应的语义是用户长按(long press)了屏幕
下图是触摸消息随时间变化的时间轴示意图:
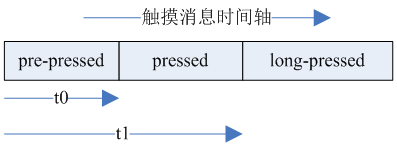
其中,t0和t1定义在ViewConfiguration类中,标识了tap和longpress的超时时间,定义如下:
/**
* Defines the duration in milliseconds we will wait to see if a touch event
* is a tap or a scroll. If the user does not move within this interval, it is
* considered to be a tap.
*/
private static final int TAP_TIMEOUT = 115; // t0
/**
* Defines the duration in milliseconds before a press turns into
* a long press
*/
private static final int LONG_PRESS_TIMEOUT = 500; // t1
代码中实现监测的地方在View类的OnTouchEvent函数中,当View监测到ACTION_DOWN事件时,首先发送一个延迟为t0的异步消息,代码如下: case MotionEvent.ACTION_DOWN:
if (mPendingCheckForTap == null) {
mPendingCheckForTap = new CheckForTap();
}
mPrivateFlags |= PREPRESSED;
mHasPerformedLongPress = false;
postDelayed(mPendingCheckForTap, ViewConfiguration.getTapTimeout());
break;
如果在t0时间内用户释放了屏幕,即ACTION_UP事件在t0时间内发生,则本次触摸对应的是pre-pressed处理代码,语义是"用户轻触(TAP)了一下屏幕";如果用户在t1时间内释放了屏幕,那么本次操作是一个"press"操作;如果用户超过t1时间释放屏幕,则系统认为监测到了长按事件。其中处理"press"操作的代码在类CheckForTap类中,处理长按操作的代码在类CheckForLongPress类中。而处理pre-pressed的代码在ACTION_UP事件响应中,ACTION_UP事件响应中大部分代码用于处理触摸的状态变化,如下所示: case MotionEvent.ACTION_UP:
boolean prepressed = (mPrivateFlags & PREPRESSED) != 0; //获取prepressed状态
if ((mPrivateFlags & PRESSED) != 0 || prepressed) { //如果是pressed状态或者是prepressed状态,才进行处理
// 如果当前view不具有焦点,则需要先获取焦点,因为我们当前处理触摸模式
boolean focusTaken = false;
if (isFocusable() && isFocusableInTouchMode() && !isFocused()) {
focusTaken = requestFocus(); // 请求获得焦点
}
if (!mHasPerformedLongPress) { // 是否处理过长按操作了,如果是,则直接返回
// 进入该代码段,说明这是一个tap操作,首先移除长按回调操作
removeLongPressCallback();
// Only perform take click actions if we were in the pressed state
if (!focusTaken) {
// Use a Runnable and post this rather than calling
// performClick directly. This lets other visual state
// of the view update before click actions start.
if (mPerformClick == null) {
mPerformClick = new PerformClick();
}
if (!post(mPerformClick)) {
performClick(); // 执行点击的处理函数
}
}
}
if (mUnsetPressedState == null) {
mUnsetPressedState = new UnsetPressedState();
}
if (prepressed) {
mPrivateFlags |= PRESSED;
refreshDrawableState();
// 发送重置触摸状态的异步延迟消息
postDelayed(mUnsetPressedState,
ViewConfiguration.getPressedStateDuration());
} else if (!post(mUnsetPressedState)) {
// If the post failed, unpress right now
mUnsetPressedState.run();
}
removeTapCallback(); // 移除tap的回调操作
}
break;
在上面的代码分析中,可以看出,整个监测过程涉及到两个Runnable对象和一个利用Handler发送异步延迟消息的函数,下面就来分析一下:
1)PostDelayed函数
该函数的主要工作是获取UI线程的Handler对象,然后调用Handler类的postDelayed函数将指定的Runnable对象放到消息队列中。
public boolean postDelayed(Runnable action, long delayMillis) {
Handler handler;
if (mAttachInfo != null) {
handler = mAttachInfo.mHandler;
} else {
// Assume that post will succeed later
ViewRoot.getRunQueue().postDelayed(action, delayMillis);
return true;
}
return handler.postDelayed(action, delayMillis);
}
2)CheckForTap类
该类实现了Runnable接口,在run函数中设置触摸标识,并刷新Drawable的状态,同时用于发送一个检测长按事件的异步延迟消息,代码如下:
private final class CheckForTap implements Runnable {
public void run() {
// 进入该函数,说明已经过了ViewConfiguration.getTapTimeout()时间,
// 即pre-pressed状态结束,宣告触摸进入pressed状态
mPrivateFlags &= ~PREPRESSED;
mPrivateFlags |= PRESSED;
refreshDrawableState(); // 刷新控件的背景Drawable
// 如果长按检测没有被去使能,则发送一个检测长按事件的异步延迟消息
if ((mViewFlags & LONG_CLICKABLE) == LONG_CLICKABLE) {
postCheckForLongClick(ViewConfiguration.getTapTimeout());
}
}
}
private void postCheckForLongClick(int delayOffset) {
mHasPerformedLongPress = false;
// 实例化CheckForLongPress对象
if (mPendingCheckForLongPress == null) {
mPendingCheckForLongPress = new CheckForLongPress();
}
mPendingCheckForLongPress.rememberWindowAttachCount();
// 调用PostDelayed函数发送长按事件的异步延迟消息
postDelayed(mPendingCheckForLongPress,
ViewConfiguration.getLongPressTimeout() - delayOffset);
}
3)CheckForLongPress类
该类定义了长按操作发生时的响应处理,同样实现了Runnable接口
class CheckForLongPress implements Runnable {
private int mOriginalWindowAttachCount;
public void run() {
// 进入该函数,说明检测到了长按操作
if (isPressed() && (mParent != null)
&& mOriginalWindowAttachCount == mWindowAttachCount) {
if (performLongClick()) {
mHasPerformedLongPress = true;
}
}
}
public void rememberWindowAttachCount() {
mOriginalWindowAttachCount = mWindowAttachCount;
}
}
public boolean performLongClick() {
sendAccessibilityEvent(AccessibilityEvent.TYPE_VIEW_LONG_CLICKED);
boolean handled = false;
if (mOnLongClickListener != null) {
// 回调用户实现的长按操作监听函数(OnLongClickListener)
handled = mOnLongClickListener.onLongClick(View.this);
}
if (!handled) {
// 如果OnLongClickListener的onLongClick返回false
// 则需要继续处理该长按事件,这里是显示上下文菜单
handled = showContextMenu();
}
if (handled) {
// 长按操作事件被处理了,此时应该给用户触觉上的反馈
performHapticFeedback(HapticFeedbackConstants.LONG_PRESS);
}
return handled;
}
分享到:
相关推荐
Android中长按弹出选项框View进行操作
Android中长连接的解决方案.docx
实现ListView上内容的长按删除功能
电信设备-处理通信系统中长时延的方法.zip
gridview单击item时选中(可多选)长按时拽拉
在Webview中长按页面识别二维码带自动解析。相信你一定会有收获!
移动端列表长按后,然后可以上下拖动进行排序,主要使用了基于h5 sortTable,然后使用了touch相关事件实现,仅支持移动端,支持安卓和苹果。
6.连接成功后返回功能模块选择列表,点击“参数设置”进入通讯相关参数配置页面(首先进入驱动配置页面),目前驱动库中只有支持Modbus TCP的驱动,后续会持续更新,用户通过在驱动库列表中长按操作将选中的驱动挑选...
Android 可以在文本框及其它文本视图中长按选择任何文字,这个操作会触发 一个文本选择模式,便于扩展选择或对选定文本进行操作。同样,该选择模式会 激活情境操作栏。Android 4.x 对选择滑块的处理很优秀,便于用户...
在TextView中长按可以复制textview的文本内容,经过验证,可以直接导入项目
但是web端实现的局限性太大,曾经也有过监听系统粘贴板,在用户点击复制的时候实现其他的逻辑,但是这样用户体验不好,所以自定义WebView中长按的弹出菜单,并在点击时返回选中文本的小控件闪亮登场┏ (^ω^)=。...
识别webview中图片,如果是二维码图片长按识别二维码
通达信指标——中长大利(副图).doc
AVS音频编码中长短窗的Matlab仿真及FPGA实现_毕业论文.pdf
高效降解菌强化处理废水中长链烷烃的效能研究,王宏,刘硕,为探究微生物菌剂对废水中长链烷烃的降解机制,由实验室分离得到的以长链烷烃为唯一碳源的降解菌株D-3和L-2,长链烷烃废水采用人工
asp.net中新闻长内容自动分页的实现方法,参考下吧
主要给大家介绍了关于shell中长命令的换行处理方法,文中通过示例代码介绍的非常详细,对大家学习或者使用shell具有一定的参考学习价值,需要的朋友们下面来一起学习学习吧
弹幕功能修复 播放器(支持普通解析/json)修复点播,游客/普通用户积分购买(会员默认关闭积分购买/用户有观影次数默认抵扣)优化用户注册限制修复播放时全屏/锁屏系统返回键恢复首页游客试看功能支持电视直播优化...